Getting Started with R
Using R without installing it
The bare minimum:
You can create vectors of numbers using this format:
x <- c(10, 12, 15, 20, 21, 22, 35, 45, 50) # click to copy -->
Then you can use R functions to find numerical and graphical summaries of the vectors (collections of numbers):
mean(x)
## [1] 25.55556
median(x)
## [1] 21
sd(x)
## [1] 14.43183
fivenum(x)
## [1] 10 15 21 35 50
stem(x)
##
## The decimal point is 1 digit(s) to the right of the |
##
## 1 | 025
## 2 | 012
## 3 | 5
## 4 | 5
## 5 | 0
hist(x)
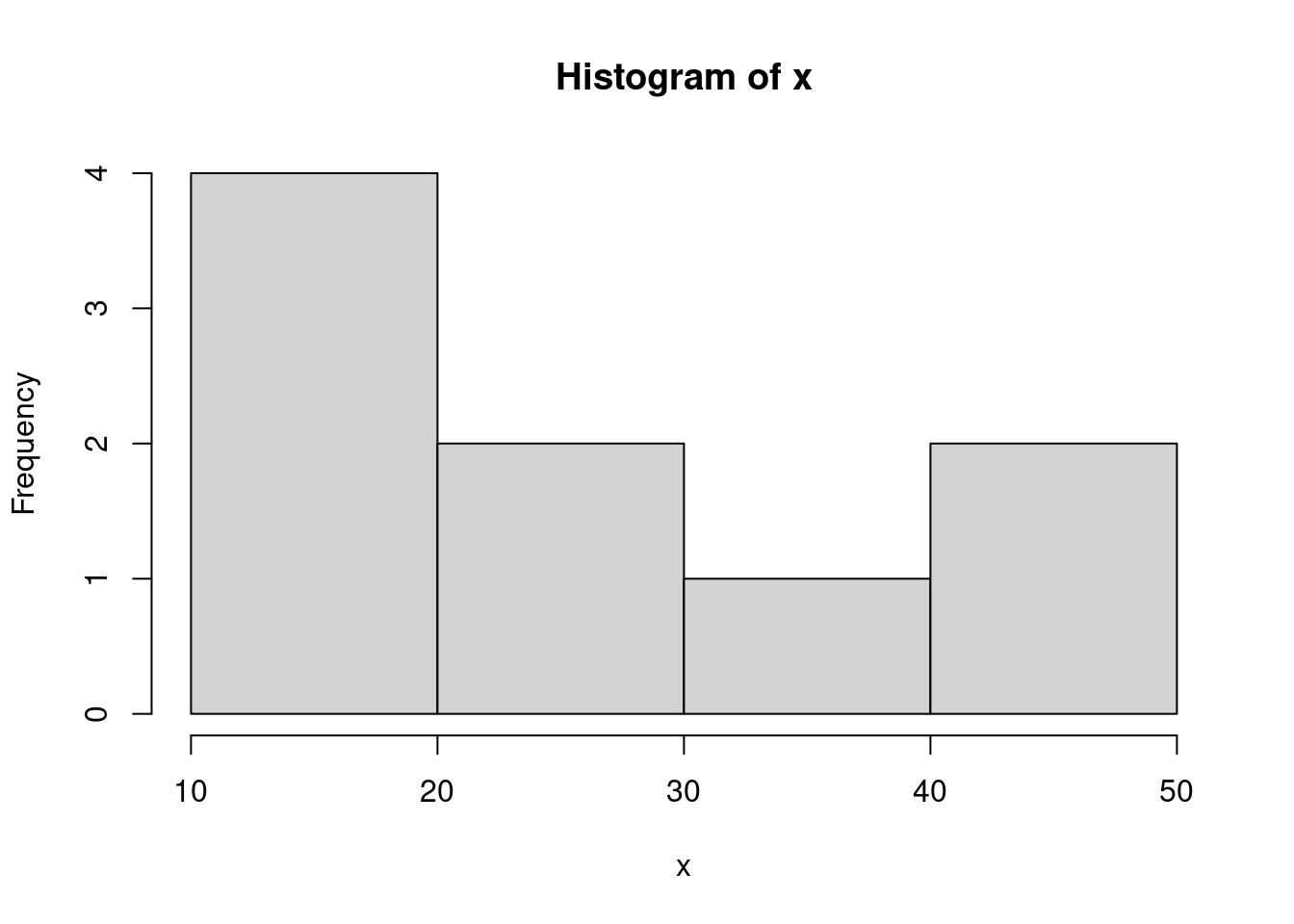
boxplot(x)
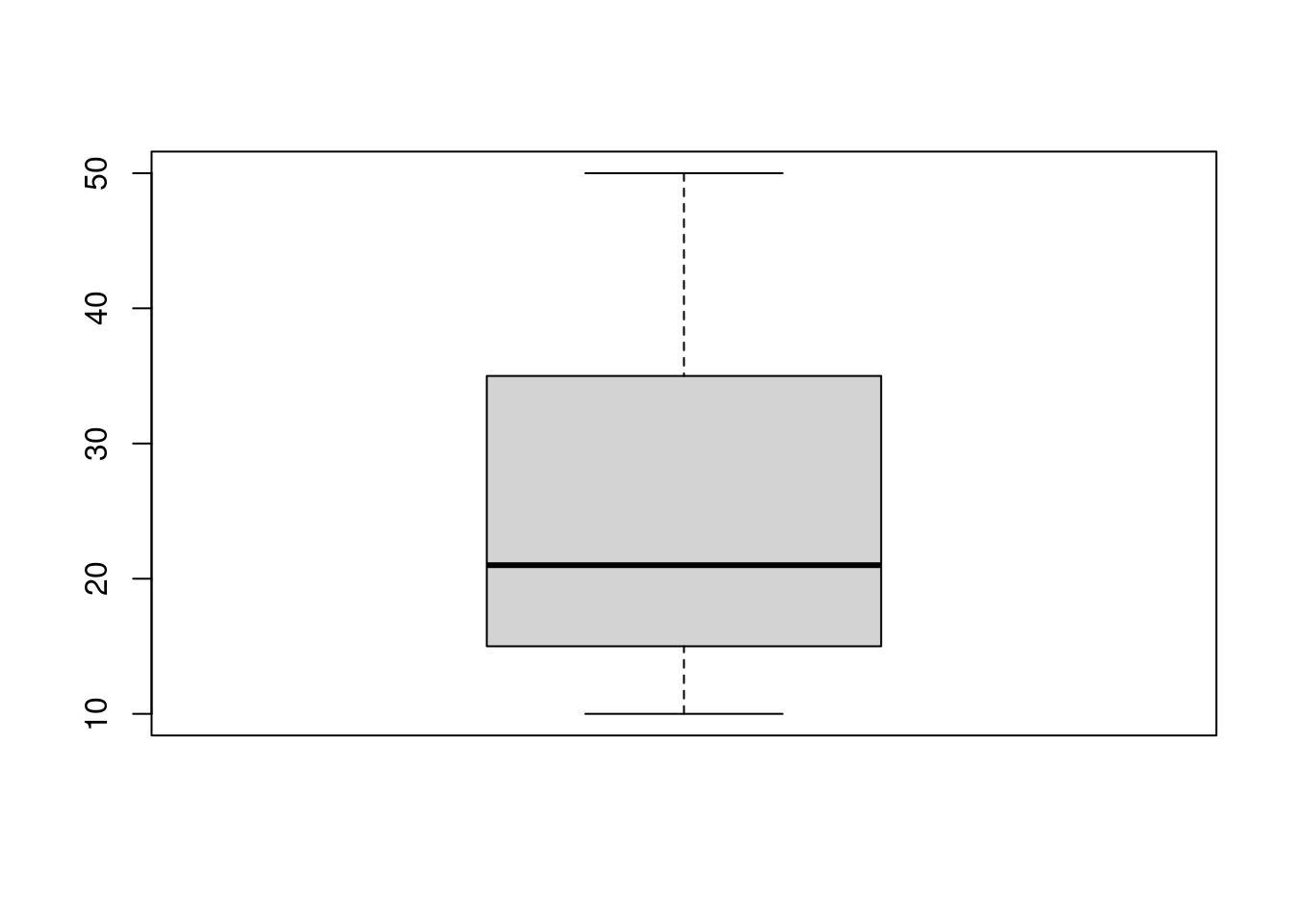
Installing R
You will need to install two applications: R and RStudio:
- R – the programming language itself – is available here:
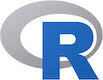
- RStudio – an integrated development environment (IDE) which makes it much easier to use R. It is optional but highly recommended. This is the app you will open to use R. Choose the free version of RStudio Desktop:
https://www.rstudio.com/products/rstudio/download/#download

Getting Started with R: Working in the Console
The first step in getting started is getting comfortable working in the RStudio console. It works like a calculator in the sense that your work is not saved. Do the following:
Quick review of material covered in the video, plus additional examples
Working in the console pane is similar to a using a calculator: each line of code is executed when you press enter. Note that your work is not saved with this approach.
Assigning a variable
Drawing a stem and leaf plot
Basic operations:
3 + 4
## [1] 7
3 - 4
## [1] -1
3 * 4
## [1] 12
3 / 4
## [1] 0.75
3^4
## [1] 81
sqrt(3)
## [1] 1.732051
Working with vectors:
x <- 1:5
x
## [1] 1 2 3 4 5
x + 10
## [1] 11 12 13 14 15
x^2
## [1] 1 4 9 16 25
sum(x)
## [1] 15
Additional resources
See the examples in Chapter 1 of Introduction to R
Creating Graphs, Saving your work
Video
Saving code as an .R file
(Also covered in video above)
Saving with this method saves only the code, not the output. Below are two methods for creating .html documents that contain both code and output:
Reading files
The easiest way to read in the data for the textbook exercises is to read it directly from the following URL as follows:
data <- read.csv("https://raw.githubusercontent.com/jtr13/1201/main/Devore9e_Final_Datasets/CSV/CH01/ex01-63.csv")
(Full URL: https://raw.githubusercontent.com/jtr13/1201/main/Devore9e_Final_Datasets/CSV/CH01/ex01-63.csv
)
Note that data
is a data frame:
head(data)
## noise
## 1 55.3
## 2 55.3
## 3 55.3
## 4 55.9
## 5 55.9
## 6 55.9
To convert it to a vector use:
noise <- data$noise
Now you can use the same functions as described above:
boxplot(noise, horizontal = TRUE)
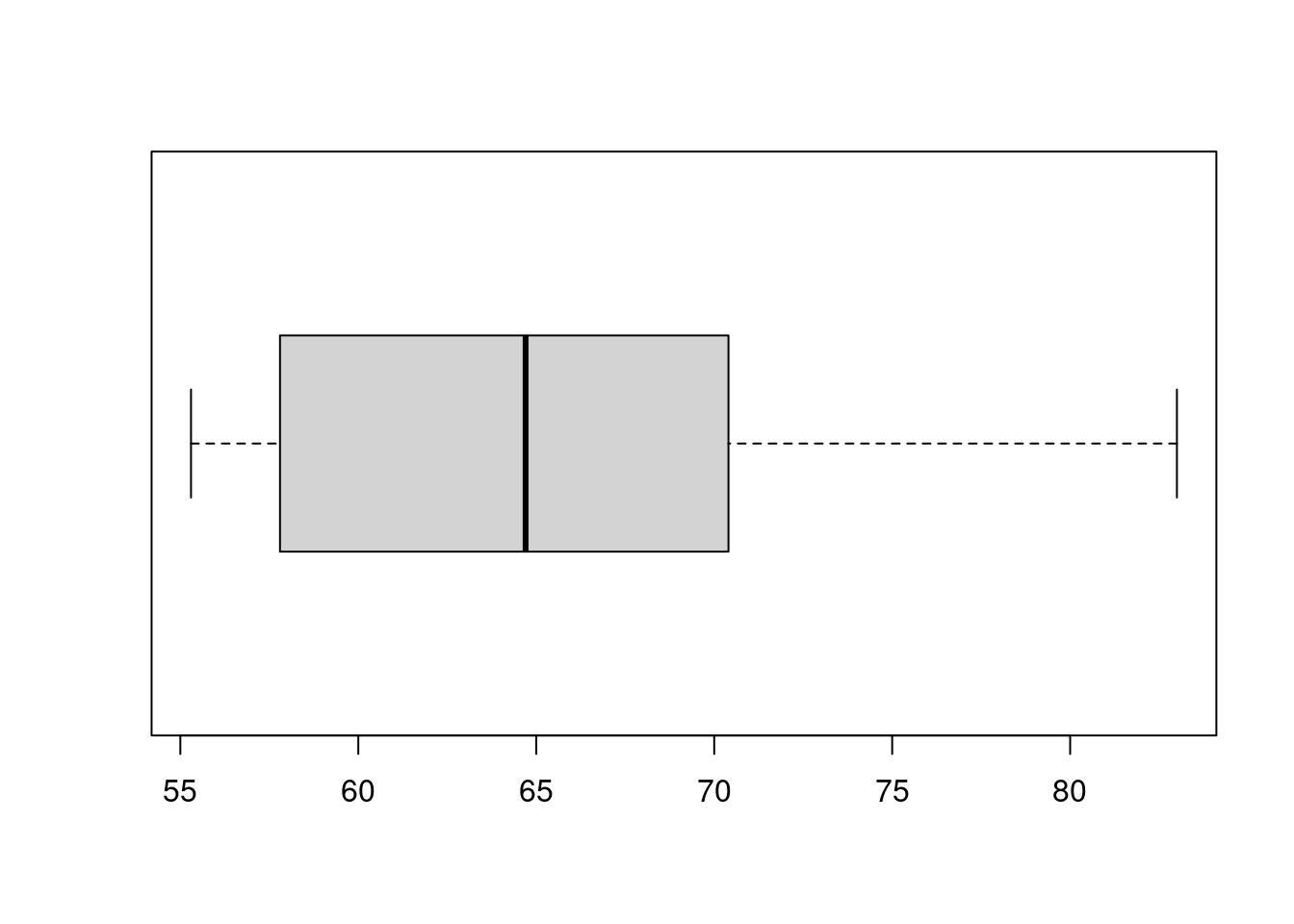